Dynamically Ignoring Unity Unit Tests the Fast Way
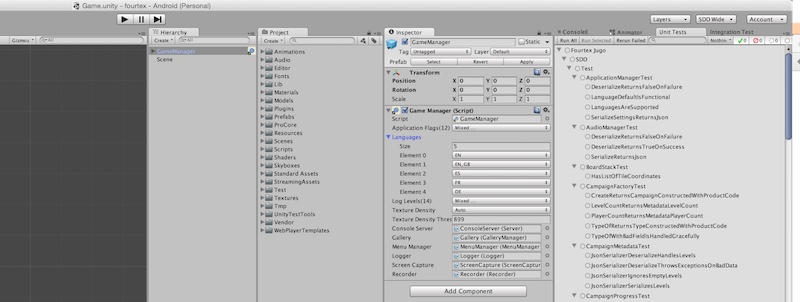
You can statically ignore Unity unit tests by applying the [Ignore]
attribute. That works fine if you only have a few work-in-progress tests to ignore. What about the use case where you have hundreds of tests that need to be ignored? This use case is very common when you have a single code framework that supports multiple games on multiple platforms.
Here at Salty Dog, we’ve tried a couple of different methods to dynamically ignore unit tests. We've tried the slow way using Assert.Ignore
and a faster method using compiler defines.
The example code snippets below are from our multiple product code base used by our Fourtex products.
The Slow Approach: Assert.Ignore
This approach executes the [Setup]
method for each test class before the test class is ignored. Test time really adds up when you have good test coverage.
An additional downside to this approach is that filtering by Assert.Ignore
is not implemented for dynamically ignored tests. You can end up with hundreds of ignored tests in the IDE and no way to hide them.
Here's our example code.
…
using SDD.Players;
using SDD.Campaigns;
using SDD.Campaigns.Zen;
namespace SDD.Test.Zen {
[TestFixture]
[Category("Campaigns")]
public class CampaignTest : UnitTest {
private Campaign campaign;
[SetUp]
public void Before() {
if (Settings.ProductCode != ProductCode.Zen) {
Assert.Ignore("These tests are for 'ProductCode.Zen'");
}
campaign = new MainCampaign();
}
…
The Fast Approach: Compiler Defines
Wrap the [Ignore]
attribute in compiler defines. This method is filterable in the Unity IDE. Better and Faster! Wee!
…
using SDD.Players;
using SDD.Campaigns;
using SDD.Campaigns.Zen;
namespace SDD.Test.Zen {
#if !SDD_PRODUCT_ZEN
[Ignore]
#endif
[TestFixture]
[Category("Campaigns")]
public class CampaignTest : UnitTest {
private Campaign campaign;
[SetUp]
public void Before() {
campaign = new MainCampaign();
}
…
How do you handle this? Tweet me. See below.
Fourtex Jugo
More Unity Shortcut Keys